How can I prepare for coding interviews while still learning
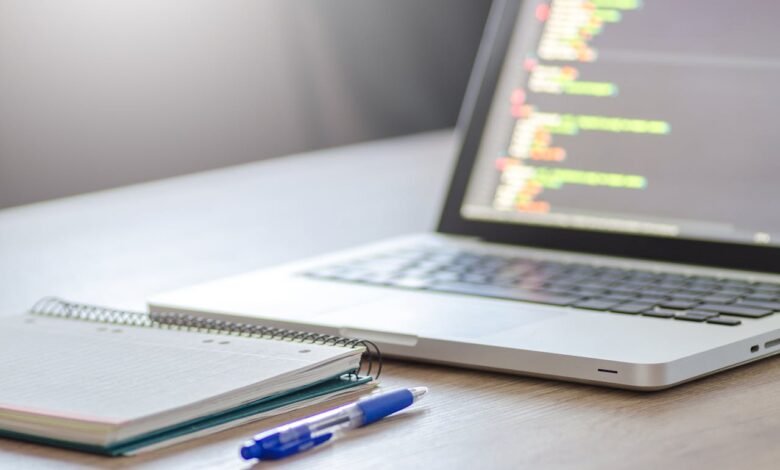
Preparing for coding interviews while still learning can be a challenging but rewarding process. This comprehensive guide will help you navigate this journey effectively, providing strategies, resources, and tips to enhance your coding skills and interview readiness.
Understanding the Coding Interview Landscape
Coding interviews are a crucial part of the hiring process for software engineering positions. They typically involve solving algorithmic problems, discussing system design, and demonstrating your coding skills in real-time. While these interviews can be daunting, especially for those still in the learning phase, with the right preparation, you can significantly improve your chances of success.
Developing a Solid Foundation
Master the Fundamentals
Before diving into complex algorithms and data structures, ensure you have a strong grasp of programming fundamentals:
- Programming Language Proficiency: Choose a language you’re comfortable with and master its syntax and core concepts. Popular choices include Python, Java, C++, and JavaScript1.
- Basic Data Structures: Understand arrays, linked lists, stacks, queues, trees, and hash tables.
- Algorithms: Familiarize yourself with sorting, searching, and basic graph algorithms.
- Time and Space Complexity: Learn to analyze and optimize your code’s efficiency.
Focus on Problem-Solving Skills
Coding interviews are not just about writing code; they’re about solving problems efficiently:
- Analytical Thinking: Practice breaking down complex problems into smaller, manageable parts.
- Pattern Recognition: Learn to identify common problem patterns and apply appropriate solutions.
- Pseudocode: Get comfortable with writing pseudocode before diving into actual implementation.
Structured Learning Approach
Create a Study Plan
Develop a structured study plan that balances learning new concepts with practicing coding problems:
- Set Realistic Goals: Determine how much time you can dedicate daily or weekly to interview preparation.
- Topic Prioritization: Focus on fundamental topics first before moving to more advanced concepts.
- Consistent Practice: Aim to solve at least one coding problem daily to build momentum.
Utilize Online Resources
Take advantage of the wealth of online resources available for coding interview preparation:
- Online Courses: Platforms like Coursera, edX, and Udacity offer courses on algorithms and data structures.
- Coding Platforms: LeetCode, HackerRank, and CodeSignal provide a vast array of practice problems and mock interviews3.
- Video Tutorials: YouTube channels like “Back To Back SWE” and “Tushar Roy – Coding Made Simple” offer in-depth explanations of common interview topics.
Practical Strategies for Effective Preparation
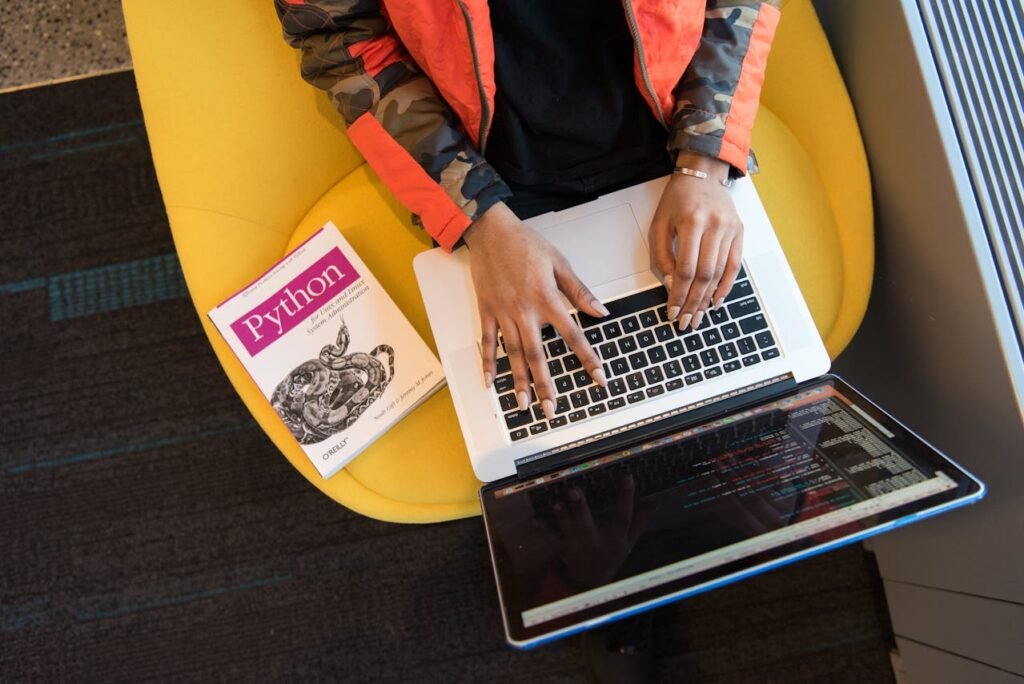
Implement the “Learn and Practice” Cycle
For each topic you study:
- Learn the Concept: Understand the theory behind the algorithm or data structure.
- Implement from Scratch: Try coding the concept without referring to resources.
- Solve Related Problems: Apply the concept to solve various coding challenges.
- Review and Optimize: Analyze your solutions and learn from others’ approaches.
Simulate Real Interview Conditions
As you progress in your preparation:
- Time-Constrained Practice: Set a timer when solving problems to mimic interview pressure.
- Mock Interviews: Use platforms like Pramp or InterviewBit for peer-to-peer mock interviews6.
- Whiteboard Practice: Practice solving problems on a whiteboard or paper to simulate non-IDE environments.
Enhancing Your Problem-Solving Approach
Develop a Systematic Approach
When tackling coding problems:
- Understand the Problem: Clarify requirements and constraints with the interviewer.
- Brainstorm Solutions: Consider multiple approaches before settling on one.
- Communicate Your Thought Process: Explain your reasoning as you work through the problem.
- Write Clean, Modular Code: Focus on writing readable and maintainable code.
- Test Your Solution: Develop test cases to verify your implementation.
Learn from Your Mistakes
After each practice session:
- Analyze Incorrect Solutions: Understand why your approach didn’t work.
- Study Optimal Solutions: Learn from more efficient or elegant solutions.
- Revisit Challenging Problems: Return to difficult problems after a few days to reinforce learning.
Balancing Learning and Interview Preparation
Integrate Interview Prep into Your Learning Process
As you continue to learn new programming concepts:
- Apply New Knowledge: Look for opportunities to use newly learned concepts in coding challenges.
- Build Projects: Develop small projects that incorporate interview-relevant topics.
- Contribute to Open Source: Gain real-world coding experience while preparing for interviews.
Stay Updated with Industry Trends
Keep abreast of current technologies and practices:
- Follow Tech Blogs: Read blogs like TechCrunch or Hacker News to stay informed about industry developments.
- Attend Coding Meetups: Participate in local coding meetups or online webinars to learn from peers and experts.
- Explore Company Tech Blogs: Many tech companies share insights about their engineering practices and interview processes.
Developing Soft Skills for Interviews
Enhance Communication Skills
Effective communication is crucial in coding interviews:
- Practice Explaining Code: Regularly explain your code and thought process to others or even to yourself.
- Engage in Technical Discussions: Participate in coding forums or discussion groups to articulate technical concepts clearly.
- Learn to Ask Clarifying Questions: Don’t hesitate to seek clarification during interviews when needed.
Cultivate a Growth Mindset
Approach your preparation with a positive attitude:
- Embrace Challenges: View difficult problems as opportunities to learn and grow.
- Learn from Feedback: Be open to constructive criticism and use it to improve.
- Persist Through Setbacks: Don’t get discouraged by initial failures; use them as learning experiences.
Leveraging Coding Interview Resources
Books for In-Depth Study
Supplement your online learning with comprehensive books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: A classic resource covering a wide range of interview topics1.
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein: For a deep dive into algorithmic concepts.
- “The Algorithm Design Manual” by Steven S. Skiena: Offers practical approaches to algorithm design.
Online Platforms for Practice
Utilize various online platforms to diversify your practice:
- LeetCode: Offers a vast collection of coding problems with difficulty ratings and company tags5.
- HackerRank: Provides a mix of algorithmic challenges and skill-based certifications.
- CodeSignal: Features company-specific assessments and practice tests.
- InterviewBit: Offers a structured learning path with curated problems and mock interviews10.
Tailoring Your Preparation to Specific Companies
Research Company-Specific Interview Processes
Different companies have varying interview styles:
- Google: Focus on algorithmic problem-solving and system design.
- Amazon: Emphasizes leadership principles alongside coding skills.
- Facebook: Concentrates on coding efficiency and optimization.
- Microsoft: Balances coding questions with design and behavioral aspects.
Practice Company-Specific Questions
Many platforms offer company-tagged questions:
- Use LeetCode’s Company Tags: Filter problems by companies that frequently ask them.
- Explore Glassdoor: Read interview experiences shared by previous candidates11.
- Participate in Company-Specific Coding Contests: Some companies host coding competitions that can give you insight into their interview style.
Maintaining Work-Life Balance During Preparation
Set Realistic Expectations
Balance your interview preparation with other responsibilities:
- Create a Sustainable Schedule: Allocate time for study, work, and personal life.
- Quality Over Quantity: Focus on understanding concepts thoroughly rather than solving a large number of problems superficially.
- Take Regular Breaks: Incorporate short breaks and days off to prevent burnout.
Stay Healthy and Motivated
Physical and mental well-being are crucial for effective learning:
- Exercise Regularly: Physical activity can improve cognitive function and reduce stress.
- Get Adequate Sleep: Ensure you’re well-rested to maintain focus during study sessions.
- Celebrate Small Wins: Acknowledge your progress to stay motivated throughout the preparation process.
Preparing for Different Types of Coding Interviews
Technical Phone Screens
Often the first step in the interview process:
- Practice Coding in a Simple Text Editor: Familiarize yourself with coding without IDE support.
- Improve Verbal Communication: Practice explaining your thought process clearly over the phone.
- Prepare Your Environment: Ensure you have a quiet space and a reliable internet connection for the call.
On-Site Coding Interviews
Typically involve more in-depth problem-solving:
- Whiteboard Coding: Practice writing code on a whiteboard or paper.
- System Design Questions: For more senior positions, study system design principles and practice designing scalable systems.
- Behavioral Questions: Prepare stories that demonstrate your problem-solving skills and teamwork.
Take-Home Coding Assignments
Some companies prefer project-based assessments:
- Time Management: Learn to scope and complete projects within given time constraints.
- Code Quality: Focus on writing clean, well-documented, and testable code.
- Follow Instructions Carefully: Pay attention to all requirements and submission guidelines.
Continuous Improvement and Learning
Reflect on Your Progress
Regularly assess your preparation:
- Track Solved Problems: Keep a log of problems you’ve solved and concepts you’ve mastered.
- Identify Weak Areas: Focus extra attention on topics you find challenging.
- Seek Feedback: If possible, get feedback from peers or mentors on your problem-solving approach.
Stay Curious and Keep Learning
The tech field is constantly evolving:
- Explore New Technologies: Stay open to learning about emerging technologies and programming paradigms.
- Read Technical Blogs: Follow blogs of respected developers and tech companies.
- Participate in Coding Challenges: Join online coding competitions to test your skills against others.
Handling Interview Day
Pre-Interview Preparation
Set yourself up for success on the day of the interview:
- Review Key Concepts: Go over important algorithms and data structures.
- Prepare Your Setup: Ensure your computer, internet, and any required software are working properly.
- Dress Appropriately: Even for virtual interviews, dress professionally to set the right mindset.
During the Interview
Stay calm and focused:
- Listen Carefully: Pay close attention to the problem statement and any hints provided.
- Think Aloud: Share your thought process as you work through the problem.
- Ask Questions: Don’t hesitate to seek clarification if something is unclear.
- Manage Your Time: Keep an eye on the clock and pace yourself accordingly.
Post-Interview Actions
After the interview:
- Reflect on Your Performance: Note areas where you did well and where you could improve.
- Send a Thank-You Note: Express your appreciation for the interviewer’s time.
- Continue Practicing: Regardless of the outcome, keep honing your skills for future opportunities.
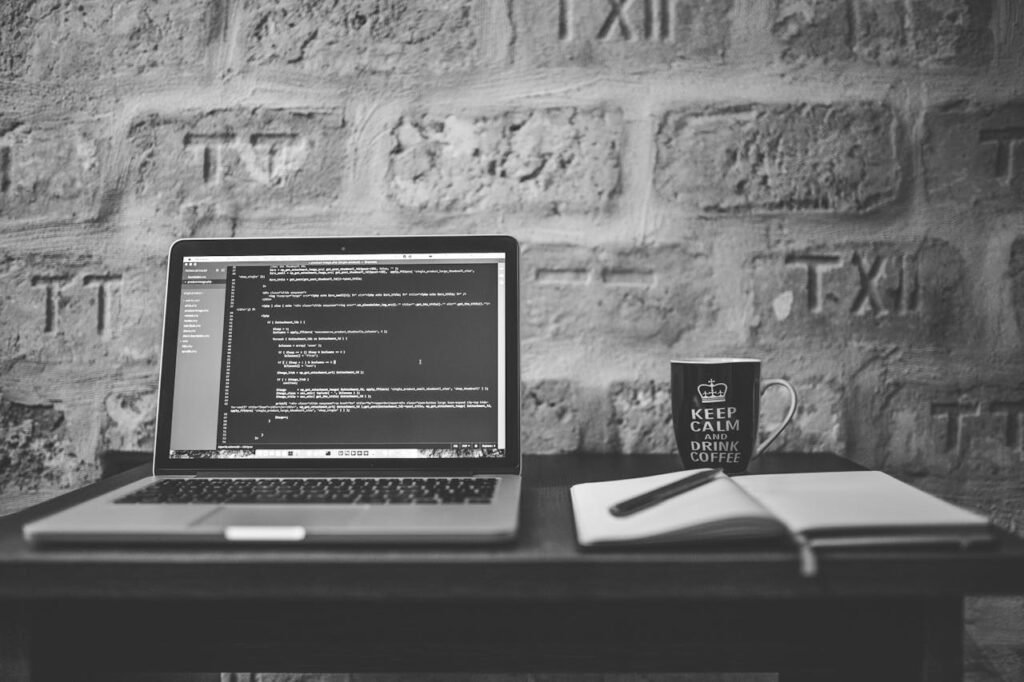
FAQs
- Q: How long should I prepare for coding interviews?
A: The duration of preparation varies depending on your current skill level and the positions you’re targeting. Generally, 3-6 months of consistent practice is recommended for thorough preparation. - Q: Is it necessary to solve all problems on platforms like LeetCode?
A: No, it’s not necessary to solve all problems. Focus on understanding core concepts and solving a diverse range of problems rather than aiming for quantity. - Q: Should I learn multiple programming languages for interviews?
A: It’s generally better to be proficient in one language rather than having superficial knowledge of many. Choose a language you’re comfortable with and master it. - Q: How important are system design questions?
A: System design questions are crucial for senior positions and at companies that work with large-scale systems. For entry-level positions, focus more on algorithmic problems. - Q: Can I use pseudocode during interviews?
A: Yes, many interviewers allow pseudocode, especially when explaining your approach. However, be prepared to write actual code as well. - Q: How do I handle nervousness during interviews?
A: Practice mock interviews, breathe deeply, and remember that it’s normal to feel nervous. Focus on the problem at hand rather than the outcome of the interview. - Q: Is it okay to ask for hints during a coding interview?
A: Yes, it’s generally acceptable to ask for hints if you’re stuck. This shows that you’re able to seek help when needed, which is a valuable skill in real work environments. - Q: How important is time complexity in coding interviews?
A: Very important. Interviewers often ask about the time and space complexity of your solutions. Practice analyzing and optimizing your code for efficiency. - Q: Should I memorize solutions to common problems?
A: It’s better to understand the underlying patterns and concepts rather than memorizing specific solutions. This allows you to adapt to new problems more effectively. - Q: How do I explain my thought process during an interview?
A: Practice thinking aloud while solving problems. Explain your approach, why you’re choosing certain data structures or algorithms, and any trade-offs you’re considering.
Comparison of Popular Coding Interview Preparation Resources
Resource | Type | Key Features | Best For |
---|---|---|---|
LeetCode | Online Platform | Large problem set, company tags, discussion forums | Comprehensive practice, company-specific prep |
HackerRank | Online Platform | Skill-based challenges, certifications | Diverse coding challenges, skill assessment |
“Cracking the Coding Interview” | Book | Comprehensive coverage, interview strategies | In-depth study, interview approach |
AlgoExpert | Online Course | Curated problem set, video explanations | Structured learning, visual learners |
InterviewBit | Online Platform | Structured learning path, mock interviews | Guided preparation, interview simulation |
Pramp | Peer-to-Peer Platform | Live mock interviews, peer feedback | Real interview practice, communication skills |
Coursera/edX Algorithms Courses | Online Courses | University-level content, certificates | Foundational knowledge, academic approach |
YouTube Tutorials | Video Content | Free, diverse topics | Visual learning, specific topic explanations |
CodeSignal | Online Platform | Company assessments, skill evaluations | Company-specific practice, skill benchmarking |
GeeksforGeeks | Website | Extensive articles, practice problems | Concept explanations, additional practice |
This table provides a quick comparison of various resources, helping you choose the ones that best fit your learning style and preparation needs. Remember, a combination of different resources often yields the best results in preparing for coding interviews.
In conclusion, preparing for coding interviews while still learning is a challenging but achievable goal. By following a structured approach, utilizing various resources, and maintaining consistent practice, you can significantly improve your coding skills and interview performance. Remember that the journey of learning and improvement is ongoing in the field of software development. Stay curious, persistent, and open to feedback, and you’ll be well on your way to success in your coding interviews and future career.